Developing AngularJS and Rails applications is our favorite way to build web applications at AmberBit. Why Angular? Because it allows us to finally separate user interface from Rails back-end. The promise of building an API along with your application is also much easier to meet when combining both frameworks. Learn how to integrate views in those two.
This post is not an introduction to setting up Rails and AngularJS app, if you are looking for one, check out this article on ShellyCloud.
AngularJS templates
Templates in AngularJS roughly correspond to views in Rails. By default, they are HTML files, enhanced with AngularJS expressions and directives.
view.html:
<div ng-controller="CompanyController">
<input type="text" ng-model="company.name">
<button ng-submit="addCompany()">
Add
</button>
</div>
You could put your “view.html” file in public/ directory and it will work just fine (provided you don’t care about cache control for example).
Writing HTML by is not fun and we already use framework that comes with HAML or Slim integration. How do we exploit that?
First, you need to put your templates in asset pipeline. We like to put them in app/assets/templates. Then, in your JavaScript files with AngularJS code, put “depend_on_asset” directive at the top and use “asset_path” in your router to load template:
application.js.coffee.erb:
#= depend_on_asset "view.html"
...
angular.module("myApp")
.config ["$routeProvider", "$locationProvider", ($routeProvider, $locationProvider) ->
$routeProvider
.when("/",
templateUrl: '<%= asset_path("view.html") %>'
)
Reload your application and you’re ready to go: your ‘view.html’ will be loaded from assets pipeline. It will also be appended with digest hash, which means the template will get refreshed after each modification in development or deployment to production.
Writing AngularJS templates HAML, Slim
You can use HAML, Slim or any other tilt-compatible language to write your templates in. Simply appending .haml or .slim extension will, however, not do the trick this time.
In order to get your AngularJS templates processed by HAML/Slim, you need to create initializer in Rails application:
config/initializers/angular_assets.rb:
# For Slim, remember also to add gem to Gemfile
Rails.application.assets.register_engine('.slim', Slim::Template)
# For HAML or other Tilt-compatible engine, use Tilt's adapters
Rails.application.assets.register_engine('.haml', Tilt::HamlTemplate)
Now we can rename our template file to app/assets/templates/view.html.slim and use shorter version of it:
div ng-controller="CompanyController"
input type="text" ng-model="company.name"
button ng-submit="addCompany()"
| Add
Yay, we just integrated Rails assets pipeline AngularJS templates in one web application. That’s a good company!
More info
- http://gaslight.co/blog/4-lessons-learned-doing-angular-on-rails
- https://github.com/rtomayko/tilt
- https://shellycloud.com/blog/2013/10/how-to-integrate-angularjs-with-rails-4
- https://leanpub.com/angularjs-rails
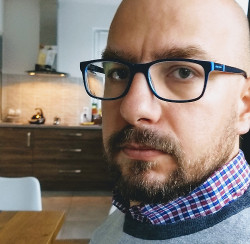
Post by Hubert Łępicki
Hubert is partner at AmberBit. Rails, Elixir and functional programming are his areas of expertise.
poplokusgafka www.yandex.ru